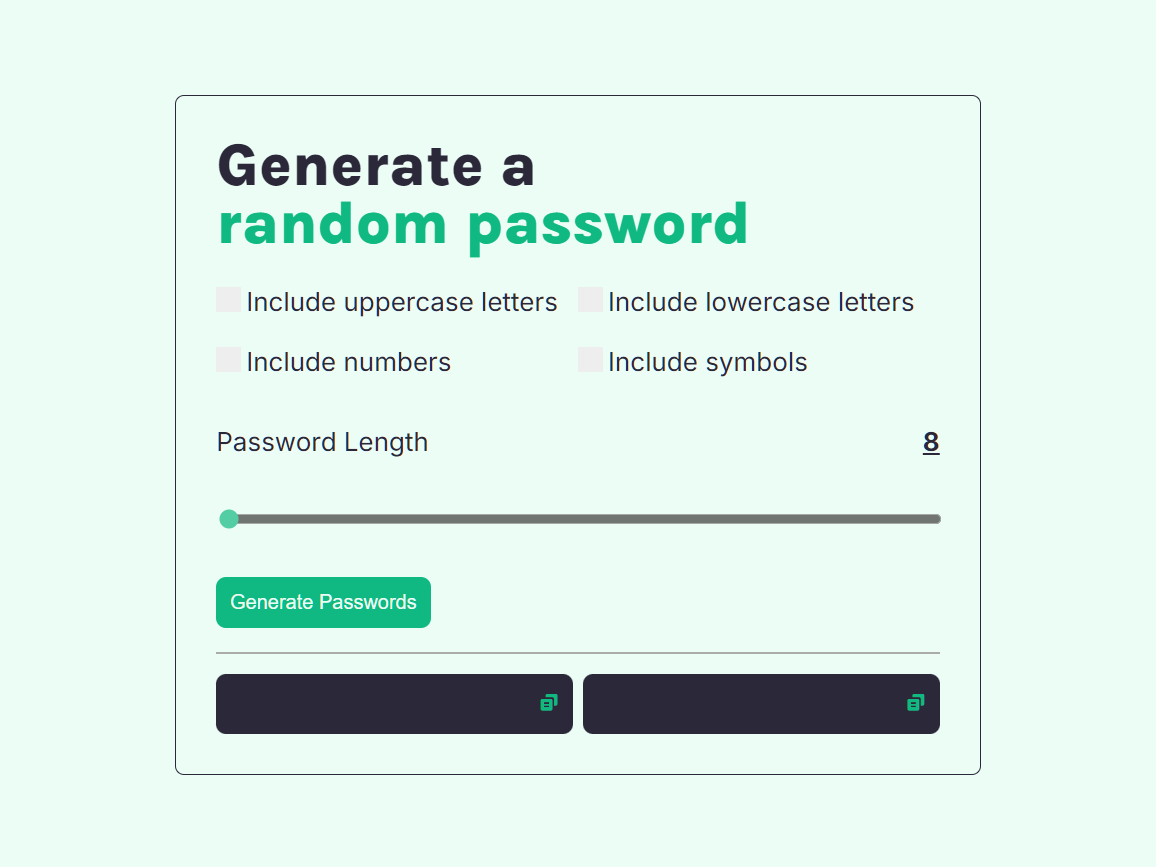
The password generator project is part of the practice time of the "Learn JavaScript" module of Scrimba
This is a part of my basic JavaScript practice specifically on the use of DOM Manipulation, arrays, loops, 'if' statement, Math floor, andMath random().
On initial build, the generator will give a randomize set of characters (uppercase, lowercase, numbers, and symbols combined) and also, default password length is limited to 15 characters. However, I decided to give a flexibility so I put an option to select what set of characters they want to be included on the generated password and in addition, range selector with a minimum of 8, and maximum of 30 characters.

First, the checkbox input is being used to select which set of characters the user wants. The "if" statement will return the value which set of characters are selected. In addition, the .concat()
method is being used to be able to combine one set of characters with another.
function retrieveChar() {
let characters = []
if (upperBox.checked) {
characters = characters.concat(upperCASE)
} if (lowerBox.checked) {
characters = characters.concat(lowerCASE)
} if (symbolBox.checked) {
characters = characters.concat(symb0ls)
} if (numB0x.checked) {
characters = characters.concat(numer1c)
}
return characters
}
Second, to make the range selector working, I made a function updateLength
wherein the length of password will depend on the range that the user will set.
// Initial password length
let passwordLength = 8
// Password length slider
function updateLength(){
passwordLength = slid3r.value
// Indicate the password length
rangeValue.textContent = passwordLength
}
As you can see from the snippet, the initial value of the password length is 8, and depending on the user, the updated number will be given based on the selected value range. The textContent
property allows the user to see the how many characters does it need to generate in the string.
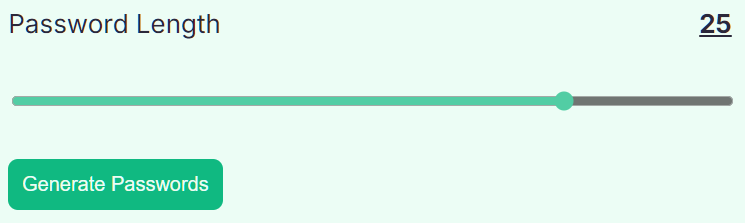
Once the characters and range has been set, the "Generate Passwords" button will trigger a function that will generate a random character. To make the randomizer work, the Math floor(Math random()...)
methods are being used.
You may be wondering why Math floor and Math random is being used together? The main purpose of Math floor is to return an integer without decimal places. In the case, we need a whole number (valid integer index in the case of array) to correctly access elements inside the characters array.
Now, the JavaScript loop will now come in to play. The purpose of the loop is that it will iterate or keep on getting random characters depending on the length value set by the user, and then it concatenates the characters to form the final passwords.

As seen from the example earlier, we have selected lowercase and numbers only to be included in the set with the length of 25 characters. The final result of generated passwords are now displayed and can be copied right away.
The "copy" function has the navigate.clipboard.writeText
property - this allows the user to copy the input value given by clicking the copy icon beside the text input.
With this project, I have learned the basic concepts of JavaScript and how it can be used to manipulate the DOM and create interactive elements on the web page.