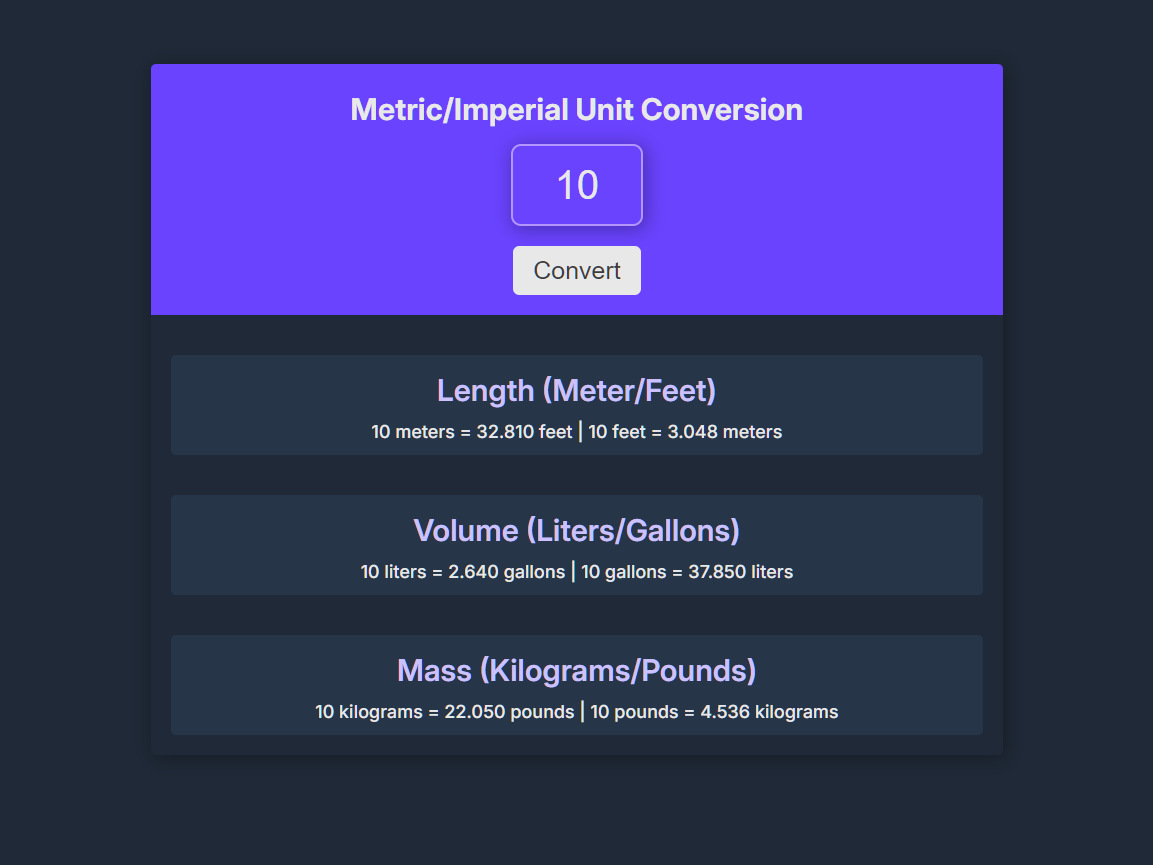
The unit converter project is part of the practice time of the "Learn JavaScript" module of Scrimba
This is a part of my basic JavaScript practice specifically on the use of event listeners, template literals, and toFixed method.
The "Convert" button has an event listener in which when clicked, it triggers a function to convert the value entered in the number input. However, the value being entered inside the input is a string so we used .parseFloat
in the script, to convert the string into a number and enable the numerical operations to proceed.
The input value is assigned to a constant variable named `baseNum` - this variable will be used inside the template literal string. Also, the template literal will render the initial input value, and the computed value to the desired unit of conversion.
The input value will be multiplied to the corresponding value of the respective unit of conversion. The target unit are as follows:
- Meter to Feet (1m = 3.281ft) and vice versa (1ft = 0.3048m)
- Liter to Gallon (1L = 0.264gal) and vice versa (1gal = 3.785L)
- Kilogram to Pound (1kg = 2.205lb) and vice versa (1lb = 0.4536kg)
As we have mentioned earlier, there is a template literal that define strings and allow expressions (e.g. multiplying the initial value to the desired unit of conversion), and the answer to the conversion of units will be rendered through .textContent
property.
The snippet example is retrieved from the length conversion. (E.g. we convert number 10 to the length units of Meter and Feet)
// length conversion (meter to feet and vice versa)
lengthEl.textContent =
`${baseNum} meters = ${(baseNum * meter2feet).toFixed(3)} feet |
${baseNum} feet = ${(baseNum * feet2meter).toFixed(3)} meters`;